Lighting models
All the lighting models described on this page use the following notation, as illustrated in the image below.

Let \(\mathbf{P}\) be the point on the surface, then:
- \(\mathbf{N}\) is the normal vector of the surface at \(\mathbf{P}\).
- \(\mathbf{L}\) is the vector from \(\mathbf{P}\) to the light source.
- \(\mathbf{V}\) is the vector from \(\mathbf{P}\) to the camera (eye).
- \(\mathbf{H}\) is the halfway vector between \(\mathbf{L}\) and \(\mathbf{V}\).
- \(\mathbf{R}\) is the reflected vector \(\mathbf{V}\) around \(\mathbf{N}\).
To find the reflection vector, we can use \(\mathbf{R} = \mathbf{V} - 2(\mathbf{V}\cdot\mathbf{N})\mathbf{N}\), where \(\mathbf{V}\cdot\mathbf{N}\) is a dot-product.
To find the halfway vector, we can use \(\mathbf{H} = \dfrac{\mathbf{L}+\mathbf{V}}{||\ \mathbf{L}+\mathbf{V}\ ||}\).
Attention
Note that all of these vectors must be normalized, which is often denoted with a hat, like \(\mathbf{\hat{N}}\).
Lambertian
Lambertian reflectance looks like an ideal "matte" or diffuse reflecting material. It is based on the angle between the light \(\mathbf{L}\) and the surface normal \(\mathbf{N}\):
where
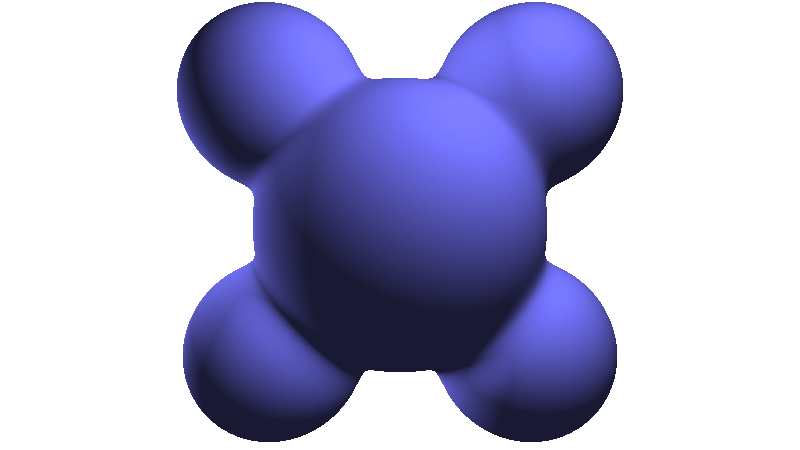
1 2 3 4 |
|
Lambertian (wrapped)
Wrapping the light can be used to fake subsurface scattering or area light.
A parameter wrap
is used which is a value between \([0, 1]\).
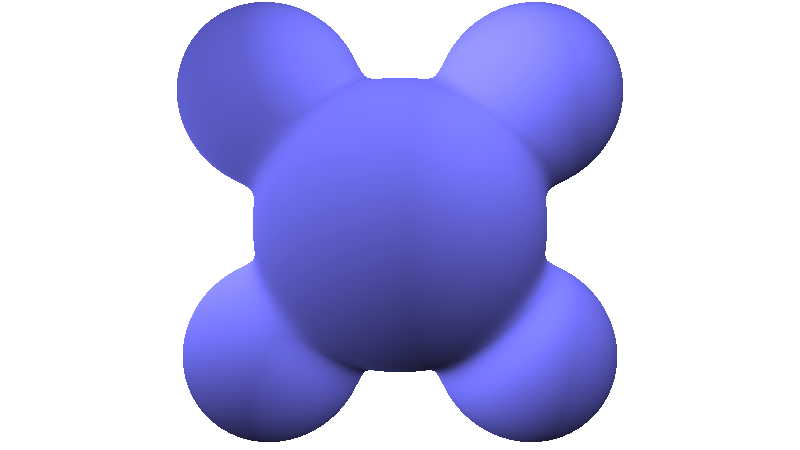
1 2 3 4 5 |
|
Phong
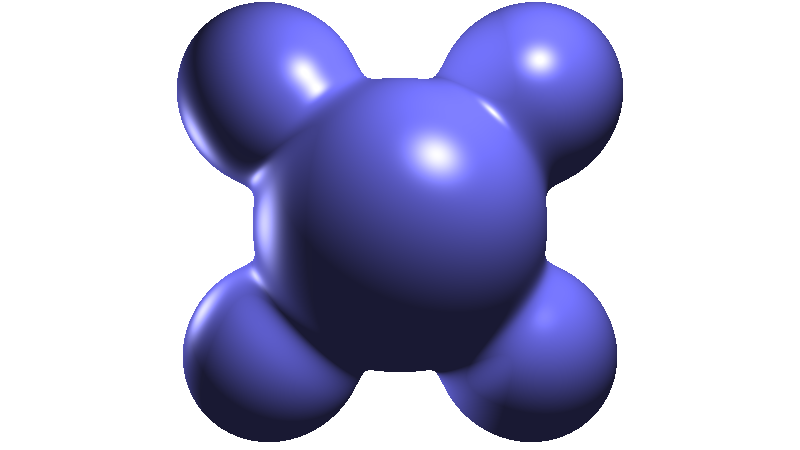
1 2 3 4 |
|
Blinn-Phong
TBA
Gaussian
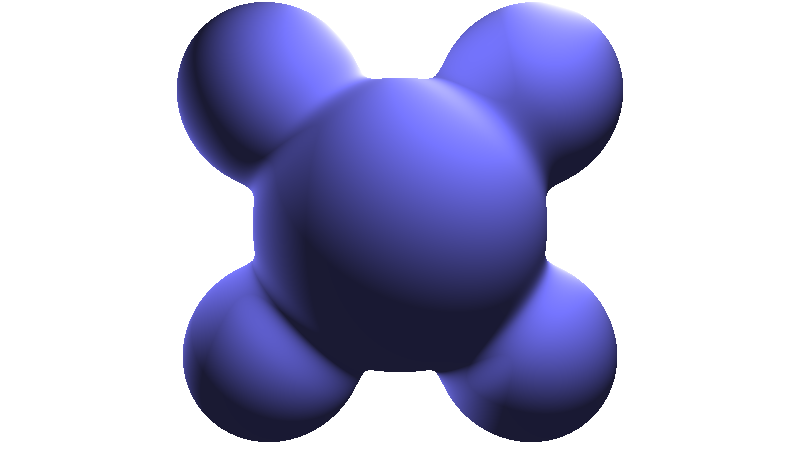
1 2 3 4 5 6 |
|
Beckmann
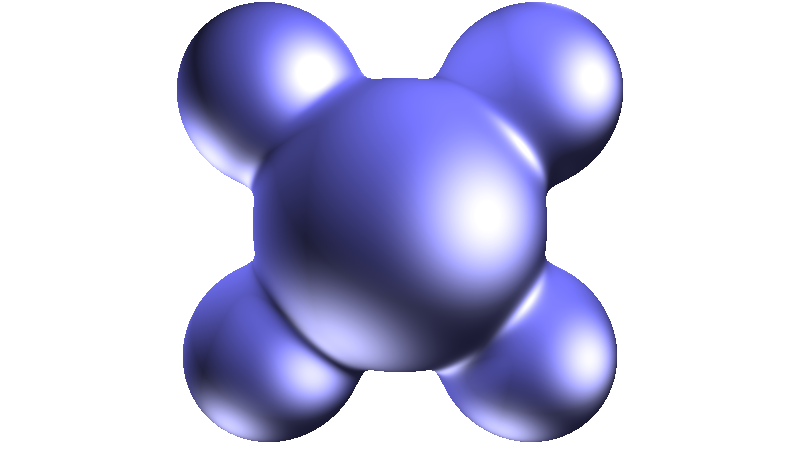
1 2 3 4 5 6 7 8 9 10 |
|
GGX
The GGX lighting model is a microfacet model for refracting through rough surfaces. It is also a model that is becoming popular for lighting in video games. [1] The GGX lighting model is derived in this paper.
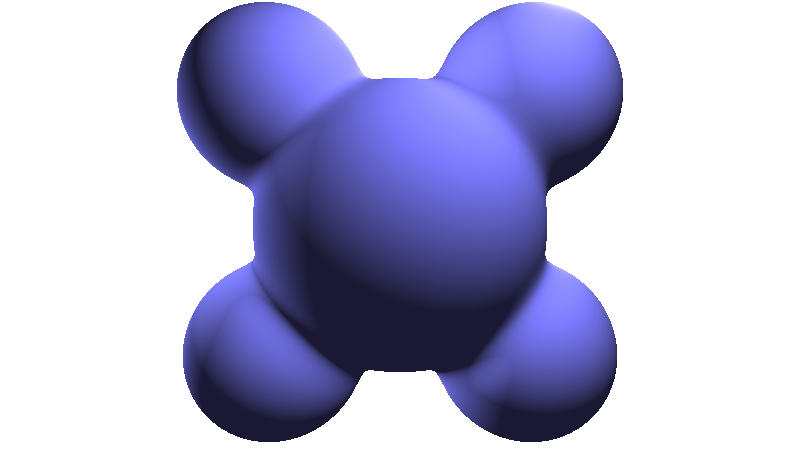
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
|
Author: John Hable
Further Reading
- Physically Based Lighting at Pixar
- Physically Based Shading at Disney
- Real Shading in Unreal Engine 4